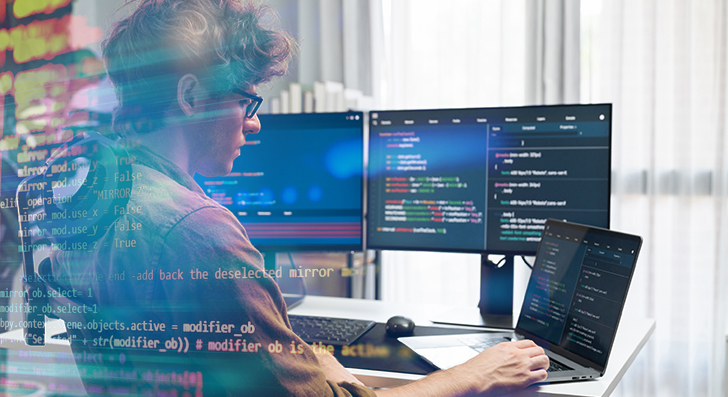
Scalability implies your application can take care of development—more buyers, far more info, and even more visitors—without breaking. For a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a transparent and sensible guideline that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability isn't anything you bolt on later—it ought to be element within your prepare from the start. A lot of purposes fall short every time they expand speedy for the reason that the original style and design can’t deal with the additional load. As a developer, you must think early about how your process will behave under pressure.
Get started by developing your architecture being flexible. Keep away from monolithic codebases where by every little thing is tightly linked. Instead, use modular design and style or microservices. These patterns break your app into scaled-down, unbiased components. Every single module or company can scale on its own without having impacting the whole technique.
Also, give thought to your databases from day 1. Will it need to have to take care of one million users or simply 100? Pick the right kind—relational or NoSQL—depending on how your facts will mature. Plan for sharding, indexing, and backups early, even if you don’t require them still.
A further important stage is to prevent hardcoding assumptions. Don’t compose code that only operates beneath recent ailments. Contemplate what would materialize In case your user base doubled tomorrow. Would your app crash? Would the databases decelerate?
Use style and design designs that guidance scaling, like concept queues or function-pushed devices. These enable your application take care of far more requests without having acquiring overloaded.
Once you Develop with scalability in mind, you're not just preparing for fulfillment—you happen to be minimizing potential head aches. A well-prepared process is simpler to maintain, adapt, and expand. It’s much better to prepare early than to rebuild afterwards.
Use the best Database
Choosing the correct databases is often a essential Component of constructing scalable programs. Not all databases are built a similar, and using the Incorrect you can sluggish you down or even bring about failures as your app grows.
Commence by comprehending your details. Could it be highly structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is a superb in shape. They're robust with interactions, transactions, and consistency. In addition they help scaling procedures like read through replicas, indexing, and partitioning to handle far more visitors and facts.
In case your information is much more flexible—like consumer exercise logs, item catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured facts and can scale horizontally a lot more conveniently.
Also, think about your examine and write designs. Will you be performing a great deal of reads with much less writes? Use caching and browse replicas. Will you be handling a hefty publish load? Take a look at databases that may take care of superior write throughput, and even celebration-centered info storage devices like Apache Kafka (for non permanent data streams).
It’s also wise to Consider forward. You might not have to have advanced scaling attributes now, but selecting a databases that supports them usually means you won’t need to switch later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your knowledge determined by your obtain styles. And usually keep track of database efficiency as you develop.
In brief, the correct database depends upon your app’s structure, velocity wants, And the way you anticipate it to develop. Consider time to pick sensibly—it’ll help you save many issues later on.
Enhance Code and Queries
Quick code is key to scalability. As your app grows, every small hold off adds up. Badly created code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s crucial that you Construct efficient logic from the start.
Start by producing clear, straightforward code. Steer clear of repeating logic and take away everything needless. Don’t choose the most advanced Resolution if a simple a person is effective. Maintain your functions small, targeted, and straightforward to check. Use profiling tools to uncover bottlenecks—spots exactly where your code usually takes also long to operate or employs an excessive amount of memory.
Future, examine your databases queries. These usually gradual items down more than the code by itself. Make sure Every single question only asks for the info you actually will need. Steer clear of Pick out *, which fetches every thing, and as a substitute choose precise fields. Use indexes to hurry up lookups. And stay away from accomplishing too many joins, Specially across massive tables.
If you recognize a similar information currently being asked for again and again, use caching. Retailer the final results temporarily making use of instruments like Redis or Memcached so you don’t should repeat expensive operations.
Also, batch your database operations any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Remember to check with large datasets. Code and queries that function fantastic with one hundred data could crash every time they have to handle 1 million.
In brief, scalable apps are quickly more info apps. Maintain your code restricted, your queries lean, and use caching when desired. These steps help your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it's got to handle more users and more visitors. If every thing goes by just one server, it can promptly turn into a bottleneck. That’s the place load balancing and caching are available in. These two resources assist keep your application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic across a number of servers. As an alternative to a single server carrying out all of the work, the load balancer routes buyers to distinctive servers based upon availability. This implies no single server receives overloaded. If just one server goes down, the load balancer can ship traffic to the Many others. Tools like Nginx, HAProxy, or cloud-centered remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing facts briefly so it can be reused promptly. When consumers request the exact same details again—like an item web page or a profile—you don’t need to fetch it through the database when. It is possible to serve it through the cache.
There are two prevalent kinds of caching:
one. Server-side caching (like Redis or Memcached) outlets info in memory for speedy accessibility.
two. Customer-side caching (like browser caching or CDN caching) merchants static files near to the person.
Caching decreases databases load, improves pace, and makes your app extra effective.
Use caching for things which don’t alter generally. And always be certain your cache is up to date when facts does alter.
Briefly, load balancing and caching are simple but effective applications. Together, they help your application handle a lot more people, stay quickly, and Get well from complications. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you may need instruments that permit your application develop very easily. That’s wherever cloud platforms and containers are available. They offer you flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and providers as you may need them. You don’t should obtain components or guess future capacity. When traffic increases, you are able to include a lot more assets with just some clicks or mechanically applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and protection equipment. You'll be able to give attention to creating your app instead of running infrastructure.
Containers are A further critical Software. A container offers your app and every thing it needs to operate—code, libraries, options—into a single unit. This can make it effortless to move your app involving environments, from the laptop to the cloud, devoid of surprises. Docker is the most well-liked Instrument for this.
Once your application employs several containers, tools like Kubernetes assist you deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of your application crashes, it restarts it instantly.
Containers also make it very easy to separate aspects of your app into products and services. It is possible to update or scale components independently, which happens to be great for overall performance and trustworthiness.
In brief, applying cloud and container equipment means it is possible to scale quick, deploy quickly, and recover speedily when problems come about. If you would like your application to mature without having restrictions, begin working with these tools early. They preserve time, decrease chance, and help you remain centered on setting up, not fixing.
Keep an eye on Everything
Should you don’t watch your software, you won’t know when items go Erroneous. Checking assists you see how your app is undertaking, location problems early, and make far better selections as your app grows. It’s a crucial Section of setting up scalable systems.
Begin by tracking standard metrics like CPU utilization, memory, disk House, and reaction time. These show you how your servers and services are performing. Equipment like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this details.
Don’t just observe your servers—monitor your app too. Keep an eye on how long it will take for end users to load web pages, how frequently glitches come about, and wherever they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, When your response time goes over a limit or a company goes down, you'll want to get notified straight away. This can help you correct troubles quickly, typically ahead of customers even discover.
Checking is likewise valuable once you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you could roll it back again prior to it results in authentic injury.
As your app grows, website traffic and info increase. Devoid of monitoring, you’ll miss indications of difficulty till it’s much too late. But with the best tools in position, you stay on top of things.
In brief, checking assists you keep your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about being familiar with your technique and making sure it works perfectly, even under pressure.
Closing Thoughts
Scalability isn’t only for big firms. Even small apps have to have a powerful Basis. By planning carefully, optimizing properly, and utilizing the correct instruments, you can Create applications that develop efficiently without breaking under pressure. Start off small, Feel major, and build wise.